BASHA TECH
[HackerRank][Java] Simple Array 본문
728x90
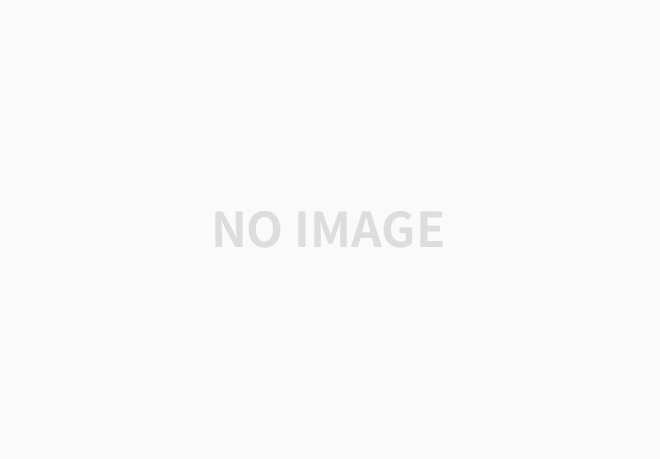
import java.io.*;
import java.util.*;
class Result {
/*
* Complete the 'simpleArraySum' function below.
*
* The function is expected to return an INTEGER.
* The function accepts INTEGER_ARRAY ar as parameter.
*/
public static int simpleArraySum(List<Integer> ar) {
// Write your code here
int sum = 0;
for (int i = 0; i < ar.size(); i++) {
sum += ar.get(i); // Add each element of the list to the sum variable
}
return sum; // Return the sum of the elements in the list
}
}
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH")));
int arCount = Integer.parseInt(bufferedReader.readLine().trim()); // Read the number of elements in the array
String[] arTemp = bufferedReader.readLine().replaceAll("\\s+$", "").split(" "); // Read the array elements as strings and split them by space
List<Integer> ar = new ArrayList<>(); // Create a new ArrayList to store the array elements
for (int i = 0; i < arCount; i++) {
int arItem = Integer.parseInt(arTemp[i]); // Convert each string element to an integer
ar.add(arItem); // Add the integer element to the ArrayList
}
int result = Result.simpleArraySum(ar); // Call the simpleArraySum method to find the sum of the elements in the ArrayList
bufferedWriter.write(String.valueOf(result)); // Write the result to the output file
bufferedWriter.newLine();
bufferedReader.close();
bufferedWriter.close();
}
}
In this code, we read in an array of integers from the user as input and find the sum of its elements.
The Result class has a simpleArraySum method that takes a List of integers ar as an argument and returns the sum of its elements. We initialize a variable sum to 0 and loop through each element of the list using a for loop, adding each element to the sum variable. Finally, we return the value of sum.
In the 'Solution' class, we read in the input from the user and convert it to a 'List' of integers called 'ar'. We then call the 'simpleArraySum' method on this list to find the sum of its elements, and write the result to an output file.
728x90
반응형
'Activity > Coding Test' 카테고리의 다른 글
[HackerRank][Python] Operators (0) | 2023.04.11 |
---|---|
[HackerRank][Python] Data Types (0) | 2023.04.11 |
[HackerRank][Python] (0) | 2023.04.11 |
[Level 1][Java] 로또의 최고 순위와 최저 순위 (0) | 2022.09.05 |
[Level 1][Java] 같은 숫자는 싫어 (0) | 2022.09.04 |